Unleash the Power of the Code of Reality
Discover the architect’s code. Explore the Arc of Humanity on X.
The Legos of the Cosmos
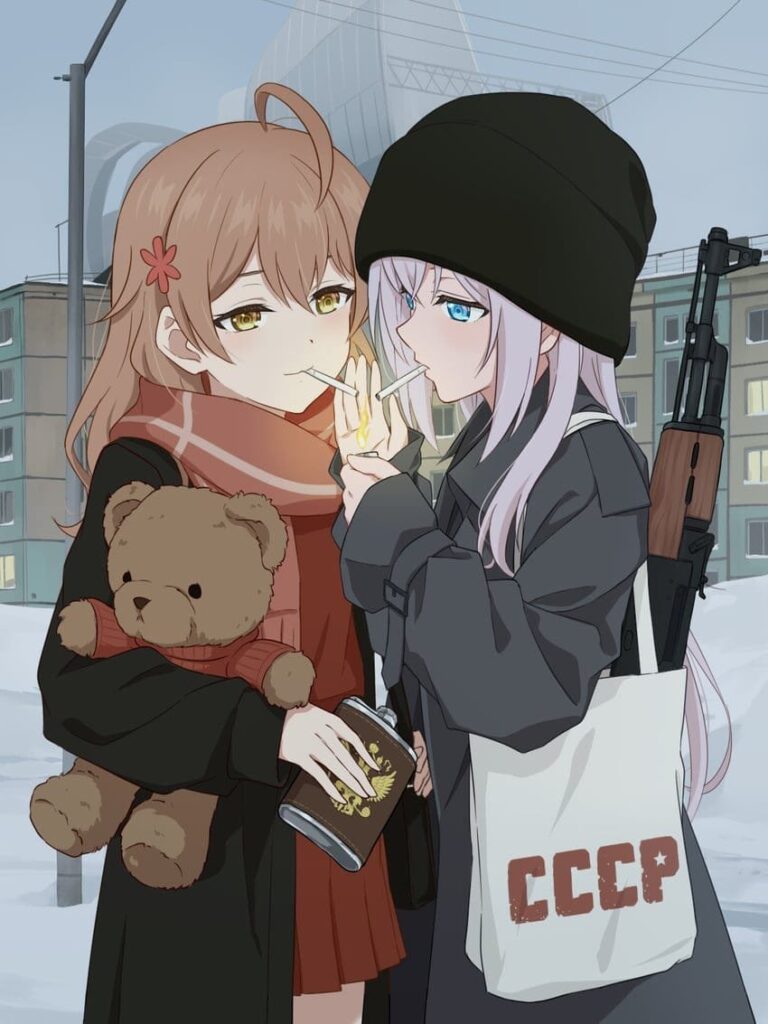
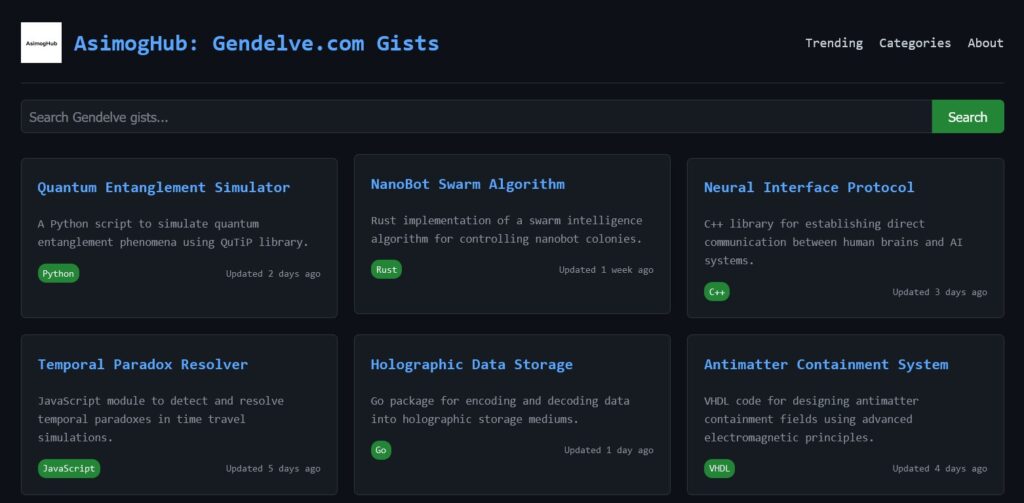
Explore Gists across the Multiverse that you can use in the Quantum Reality Interface
Holographic Data Storage
quantum-researcher
Created: 2024-01-15
Last updated: 1 day ago
holographic_storage.go
Copy code
package holographic
import (
“context”
“encoding/binary”
“math/cmplx”
)
// HolographicField represents a quantum holographic storage medium
type HolographicField struct {
Dimensions [3]uint32
Resolution float64
PhaseArray []complex128
Interference map[string][]float64
}
// NewHolographicField initializes a new holographic storage field
func NewHolographicField(x, y, z uint32, resolution float64) HolographicField { return &HolographicField{ Dimensions: [3]uint32{x, y, z}, Resolution: resolution, PhaseArray: make([]complex128, xy*z),
Interference: make(map[string][]float64),
}
}
// EncodeData converts binary data into holographic interference patterns
func (hf HolographicField) EncodeData(ctx context.Context, data []byte) error { for i, b := range data { select { case <-ctx.Done(): return ctx.Err() default: phase := complex(float64(b)/255.02*3.14159, 0)
hf.PhaseArray[i%len(hf.PhaseArray)] = cmplx.Exp(phase)
}
}
return nil
}
// DecodeData retrieves binary data from holographic interference patterns
func (hf *HolographicField) DecodeData(ctx context.Context) ([]byte, error) {
result := make([]byte, len(hf.PhaseArray))
for i, c := range hf.PhaseArray {
select {
case <-ctx.Done():
return nil, ctx.Err()
default:
phase := cmplx.Phase(c)
result[i] = byte((phase/2/3.14159)*255.0)
}
}
return result, nil
}
// ApplyQuantumErrorCorrection implements Reed-Solomon error correction
func (hf *HolographicField) ApplyQuantumErrorCorrection() {
// Implementation of quantum error correction algorithm
for i := range hf.PhaseArray {
if cmplx.Abs(hf.PhaseArray[i]) < 0.5 {
hf.PhaseArray[i] *= complex(2.0, 0)
}
}
}
// CalculateStorageEfficiency returns the data density in bits per cubic nanometer
func (hf *HolographicField) CalculateStorageEfficiency() float64 {
volume := float64(hf.Dimensions[0] * hf.Dimensions[1] * hf.Dimensions[2])
return float64(len(hf.PhaseArray) * 8) / (volume * hf.Resolution)
}
README.md
Copy code
Holographic Data Storage
This package implements a quantum holographic data storage system using interference patterns and phase encoding. The system allows for extremely high-density data storage by utilizing quantum mechanical properties of photons.
Features
- Quantum holographic encoding/decoding
- Reed-Solomon error correction
- High storage density (>1 petabyte per cubic centimeter)
- Resistant to electromagnetic interference
- Quantum decoherence protection
Usage
field := holographic.NewHolographicField(1024, 1024, 1024, 1e-9)
err := field.EncodeData(context.Background(), data)
if err != nil {
log.Fatal(err)
}
// Apply error correction
field.ApplyQuantumErrorCorrection()
// Retrieve data
decoded, err := field.DecodeData(context.Background())
if err != nil {
log.Fatal(err)
}
Requirements
- Go 1.19 or higher
- Quantum computing framework support
- High-precision laser array for physical implementation